模板引擎
◼ Thymeleaf 是新一代的服务器端 Java 模板引擎。
◼ 适用于 Web 和独立环境,能够处理 HTML,XML,JavaScript,CSS 甚至纯文本等。
◼ Thymeleaf 从一开始就支持HTML5标准。
◼ Spring4.0 推荐Thymeleaf作为前端模版引擎完全取代 JSP。
模板引擎工作流程
自然模板概念
◼ 同一个 HTML 文件,前端人员以 静态原型 方式打开,看到是静态内容。
◼ 而后端人员通过服务器运行打开时,看到是 动态数据。
◼ 自然模板实现前后端分离
静态原型
◼ 不启动服务器,直接使用浏览器预览页面:
动态运行
基本语法
常用表达式
◼ 变量表达式 ${}
◼ 选择(星号)表达式 *{}
◼ 链接表达式 @{}
(1)变量表达式:${}
◼ 使用方法:th:xx = “${}” 获取值给 th:xx
控制器代码
1 | Student student = new Student(2020001,"小明"); |
视图代码
1 | <p th:text="${count}">计数</p> |
${变量}: 获得变量值,${对象.属性}: 获得对象属性值
th:text:文本属性,用于进行文本替换
${} 一些用法
◼ 字符串连接、数学运算、布尔逻辑和三目运算等。
1 | <p th:text=" '欢迎' + ${stu.name} ">hello</p> <!-- 字符串连接 --> |
(2)选择(星号)表达式:*{}
◼ 使用方法:首先通过 th:object 获取对象,然后使用 th:xx = “{属性}” 获取对象属性值(表示 不用写对象名)。
控制器代码
1 | Student student = new Student(2019001,"小明"); |
视图代码
1 | <div th:object="${stu}" > <!-- 先在父标签中用 th:object 获得 stu 对象 --> |
(3)链接表达式:@{ }
◼ 使用方法:@{ 资源路径 }
◼ 常用于超链接和引用图片、样式、js 等静态资源
示例 1:超链接用法
◼ HTML 原生写法:
1 | <a href="/save">保存</a> |
◼ Thymeleaf 动态绑定写法:
1 | <a th:href="@{/save}">保存</a> |
◼ RESTful 风格请求:
1 | <a th:href="@{/th/edit/{id}(id=${stu.id})}">编辑</a> |
这种把参数用”/“接在 url 请求中,称为:RESTful 风格
示例 2:图片资源使用
◼ HTML 原生静态写法:
1 | <img src="/images/苏轼.jpg" > |
◼ Thymeleaf 动态绑定写法:
1 | <img th: src="@{${imgURL}}" > <!-- 即可绑定动态变量值 --> |
条件判断
◼ th:if
当条件为 true 则显示。
◼ th:unless
当条件为 false 则显示。
1 | <p th:if = "${flag}" >hello</p> |
如果 flag 值为 true,则显示 p 标签,否则不显示
1 | <p th:unless = "${flag}" >hello</p> |
如果 flag 值为 false,则显示 p 标签,否则不显示
th:switch / th:case 用法
1 | <div th:switch="${stu.id}"> |
迭代循环
◼ th:each
遍历集合,基本语法:
1 | <tr th:each="变量 : 集合"> <!-- th:each 通常写在父标签,通过遍历,动态生成多个父标签tr,tr中包含td子标签 --> |
1 | <tr th:each="变量, 状态变量 : 集合"> <!-- 通过状态变量可以获取迭代状态,比如:状态变量.index可以获得迭代序号值(从0开始) --> |
示例1:
控制器代码
1 | List<Student> stuList=new ArrayList<Student>(); |
视图代码
1 | <table> |
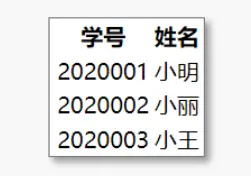
示例2:
1 | <table> |
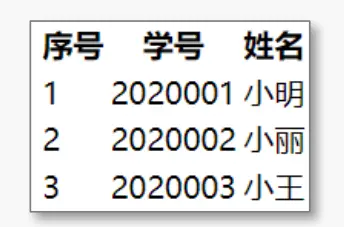
form 相关
◼ th:action
指定表单提交地址
◼ th:value
给 value 属性赋值
◼ th:field
能自动生成 id、name 和 value 属性(一次搞定三个属性)
form 表单示例
视图代码
1 | <!-- th:action:设置表单提交地址; method=post方式提交数据 --> |
综合示例
学生信息编辑和修改
项目结构
ThController 代码
1 |
|
业务功能1:列表显示
1 | // url: localhost:8080/th/ // 最后要有"/",因为该路由从属于路由组/th |
业务功能2:编辑
1 | // url: localhost:8080/th/edit/2020001 // REST风格请求 |
业务功能3:保存
1 | // url: localhost:8080/th/save 提交值为id和name post方式 |
业务功能4:删除
1 | // url: localhost:8080/th/delete/2020001 // REST风格请求 |
业务功能5:其他业务
1 | // 内部方法,外部不能访问 |
视图主要代码:index.html
1 | <div class="container"> |
视图主要代码:edit.html
1 | <div class="container"> |
界面样式:使用 Bootstrap 样式
◼ 在页面中添加 bootstrap 库:(两个视图都在 head 尾添加)
1 | <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css"> |
练习
◼ 添加学生信息创建模块。
附录
常用属性和指令
关键字 | 功能介绍 | 示例 |
---|---|---|
th:id | 替换id | <input th:id="'xxx' + ${collect.id}"/> |
th:text | 文本替换 | <p th:text="${collect.description}">description</p> |
th:utext | 支持html的文本替换 | <p th:utext="${htmlcontent}">content |
th:object | 替换对象 | <div th:object="${session.user}"> |
th:value | 属性赋值 | <input th:value = "${user.name}" /> |
th:with | 定义局部变量 | <div th:with="df='dd/MMM/yyyy HH:mm'"><div> |
th:style | 设置样式 | <div th:with="df='dd/MMM/yyyy HH:mm'"><div> |
th:onclick | 点击事件 | <td th:onclick = "'getCollect()'"></td> |
th:each | 循环迭代 | <tr th:each = "user,userStat:${users}"> |
th:if | 判断条件 | <a th:if = "${userId == collect.userId}"> |
th:unless | 和th:if判断相反 | <a th:href="@{/login} th:unless=${session.user != null}">Login |
th:href | 链接地址 | <a th:href="@{/login}" th:unless=${session.user != null}>Login</a> |
th:switch | 多路选择配合th:case | <div th:switch="${user.role}"> |
th:case | th:switch的一个分支 | <p th:case = "'admin'">User is an administrator |
th:fragment | 定义模版片段 | <div th:fragment="copy"> |
th:insert | 将片段插⼊到自己的标签体中 | <div th:insert="footer :: copy"></head> |
th:replace | 将引用的片段替换掉自己 | <div th:replace="footer :: copy"> |
th:selectd | selected选择框选中 | <option th:selected="1 == ${sex}">男 |
th:src | 选择框选中 | <img th: src="@{/img/logo.png}" > |
th:inline | 定义内联JS | <script th:inline="javascript"> |
th:action | 表单提交的地址 | <form th:action="@{/subscribe}"> |
th:remove | 删除某个属性 | <tr th:remove="all"> 1.all:删除包含标签和所有的孩子。 2.body:不包含标记删除,但删除其所有的孩子。 3.tag:包 含标记的删除,但不删除它的孩子。 4.all-but-first:删除所有包含标签的孩子,除了第一个。 5.none:什么也不做 |
th:attr | 设置标签属性,多个属性可以 用逗号分隔 | <img th:attr="src=@{/image/aa.jpg},title=#{logo}"> ,不太优雅较少使用 |